Basics:
REST stands for Representational State Transfer.
JAX-RS is a Java programming language API for exposing the Restful web services according to the REST architecture style.
Jersey is the reference implementation of JAX-RS 311 specification.
Resource is an URI which uniquely identifies the resource.
Representation is the response provided by the resource.
For instance http://developersofjava.blogspot.in/2012/04/restful-web-services-tutorial.html is the Resource.
Representation of the resource http://developersofjava.blogspot.in/2012/04/restful-web-services-tutorial.html is in HTML which will be rendered to the browser.
Restful web services works on the HTTP methods
GET- Retrieve a representation
PUT- Modify a representation
POST- Create a representation
DELETE- Delete a representation
The REST operations can be performed only on the above methods.
Java had exposed the APIs as part of the JAX-RS 311 specification. The runtime providers must implement this specification to provide the Restful web services. The Restful web services developer is only needed to be aware of the JAX-RS standards, not necessarily the details of the runtime provider of the JAX-RS.
Hello world Restful web services with Jersey(JAX-RS 311 reference implementation):
- Create a dynamic web project in your Java EE Eclipse IDE as shown in the below picture.
- Download the below shown Jersey libraries. If any of the below links did not work you can directly download them from http://jersey.java.net/
- Place the downloaded Jersey libraries in the WEB-INF/lib directory.
- Create
HelloWorldREST.java
root resource class as shown below. - Configure the web.xml file as shown like below.
- Make the web achieve and deploy into the Tomcat. For your convince i have attached the source code and war file.

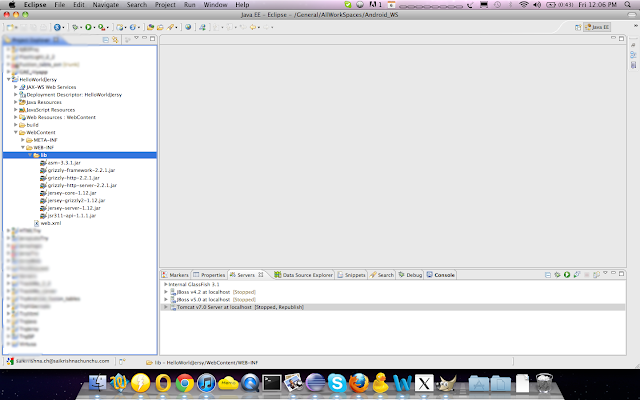
package com.sai.doj.rootresources;
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("/helloworld")
public class HelloWorldREST {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String helloWorld(){
return "Hellow world";
}
}
@path
- The relative path for this root resource class. This can be specified at the method level as well.@Produces
- The type of the representation. The method helloworld produces text output. You can produce JSON,XML and HTML etc... as well.@GET
- for the get request of the resource specified with the @path annotation, the @Get annotated method will respond.The URL to access the resource that you deployed is http://localhost:8080/HelloWorldJersy/rest/helloworld
http://localhost:8080/ - Domain where the Tomcat is is installed
HelloWorldJersy - Name of the war file or display-name
rest - where our REST services are published. This is configured in web.xml
helloworld - Name of the resource, which is specified with @path in root resource class.
If you are facing any issue in making it to work, you can comment with the necessary details in this section Jersey tutorial, i am ready to help.
1 comment:
Good Tutorial. Started learning..
Thanks .:)
Post a Comment